Bash Basics Series #3: Passing Arguments and Accepting User Inputs
Let's have arguments... with your bash scripts 😉
You can make your bash script more useful and interactive by passing variables to it.
Let me show you this in detail with examples.
Pass arguments to a shell script
When you run a shell script, you can add additional variables to it in the following fashion:
./my_script.sh var1 var2
Inside the script, you can use $1 for the 1st argument, $2 for the 2nd argument and so on.
$0 is a special variable that holds the name of the script being executed.
Let's see it with an actual example. Switch to the directory where you keep your practice bash scripts.
mkdir -p bash_scripts && cd bash_scripts
Now, create a new shell script named arguments.sh
(I could not think of any better names) and add the following lines to it:
#!/bin/bash
echo "Script name is: $0"
echo "First argument is: $1"
echo "Second argument is: $2"
Save the file and make it executable. Now run the script like you always do but this time add any two strings to it. You'll see the details printed on the screen.
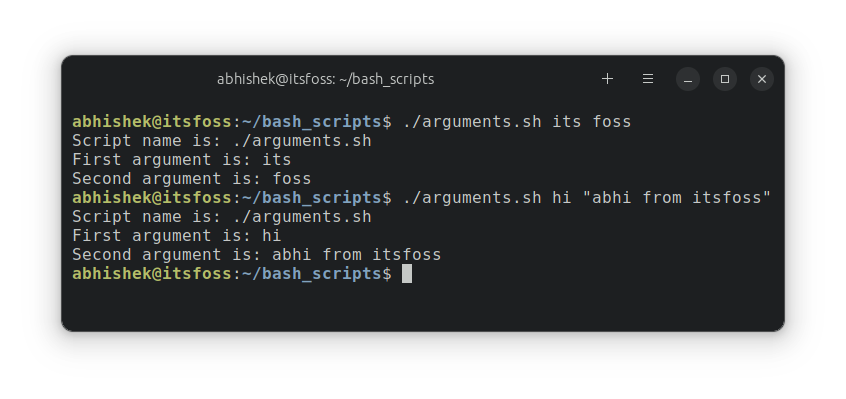
Arguments are separated by a white space (space, tab). If you have an argument with space in it, use double quotes around it otherwise it will be counted as separate arguments.
As you can see, the $0 represents the script name while the rest of the arguments are stored in the numbered variables. There are some other special variables that you may use in your scripts.
Special Variable | Description |
---|---|
$0 | Script name |
$1, $2...$n | Script arguments |
$# | Number of arguments |
$@ | All arguments together |
$$ | Process id of the current shell |
$! | Process id of the last executed command |
$? | Exit status of last executed command |
Modify the above script to display the number of arguments.
What if the number of arguments doesn't match?
In the above example, you provided the bash script with two arguments and used them in the script.
But what if you provided only one argument or three arguments?
Let's do it actually.
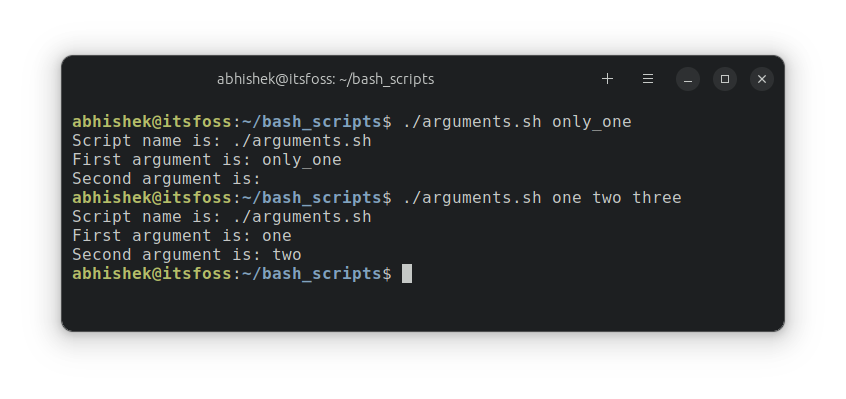
As you can see above, when you provided more than expected arguments, things were still the same. Additional arguments are not used so they don't create issues.
However, when you provided fewer than expected arguments, the script displayed empty space. This could be problematic if part of your script is dependent on the missing argument.
Accepting user input and making an interactive bash script
You can also create bash scripts that prompt the user to provide input through the keyboard. This makes your scripts interactive.
The read command provides this feature. You can use it like this:
echo "Enter something"
read var
The echo command above is not required but then the end user won't know that they have to provide input. And then everything that the user enters before pressing the return (enter) key is stored in var
variable.
You can also display a prompt message and get the value in a single line like this:
read -p "Enter something? " var
Let's see it in action. Create a new interactive.sh
shell script with the following content:
#!/bin/bash
echo "What is your name, stranger?"
read name
read -p "What's your full name, $name? " full_name
echo "Welcome, $full_name"
In the above example, I used the name
variable to get the name. And then I use the name
variable in the prompt and get user input in full_name
variable. I used both ways of using the read command.
Now if you give the execute permission and then run this script, you'll notice that the script displays What is your name, stranger?
and then waits for you to enter something from the keyboard. You provide input and then it displays What's your full name
type of message and waits for the input again.
Here's a sample output for your reference:
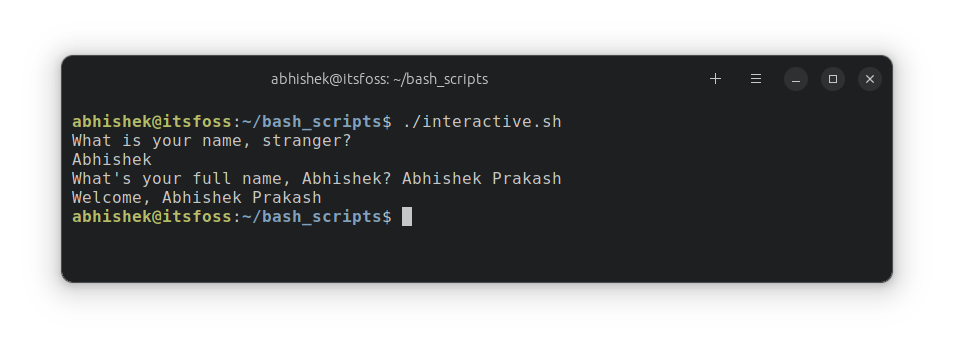
🏋️ Exercise time
Time to practice what you learned. Try writing simple bash scripts for the following scenarios.
Exercise 1: Write a script that takes three arguments. You have to make the script display the arguments in reverse order.
Expected output:
abhishek@itsfoss:~/bash_scripts$ ./reverse.sh ubuntu fedora arch
Arguments in reverse order:
arch fedora ubuntu
Exercise 2: Write a script that displays the number of arguments passed to it.
Hint: Use special variable $#
Expected output:
abhishek@itsfoss:~/bash_scripts$ ./arguments.sh one and two and three
Total number of arguments: 5
Exercise 3: Write a script that takes a filename as arguments and displays its line number.
Hint: Use wc command for counting the line numbers.
You may discuss your solution in the community.
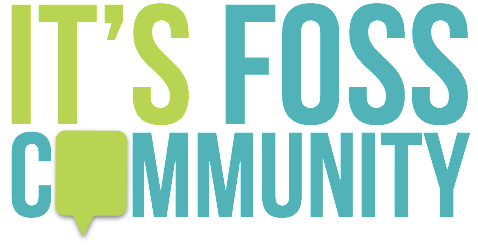
Great! So now you can (pass) argument 🙂 In the next chapter, you'll learn to perform basic mathematics in bash.
Source: It's FOSS