How to Add Comments in Bash Scripts
Adding comments in bash scripts is one of the most effective ways to keep your code clean and understandable.
You may ask why.
Let's suppose your script contains a complex regex or multiple complex blocks of codes and in that case, you can add comments so other developers or you can have an idea of what that block of code was meant to be.
Commenting out part of code also helps in debugging scripts.
In this tutorial, I will walk you through three ways to add comments in bash scripts:
- Single-line comments
- In line comments
- Multi-line comments
So let's start with the first one.
Single-line comments in bash
To add single-line comments, you have to put the hashtag (#) at the beginning of the line and write a comment.
Here's a simple example:
#!/bin/bash
# This is a comment
echo "Hello, World!"
While executing, the comments will be ignored and when I executed the above command, it looked like this:
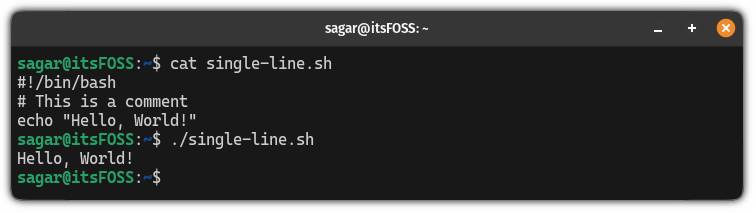
Inline comments in bash scripts
Alternatively, you can put the comment inside the code block to document what is the purpose of that specific line.
Here's a simple example:
#!/bin/bash
echo "Hello, World!" #Prints hello world
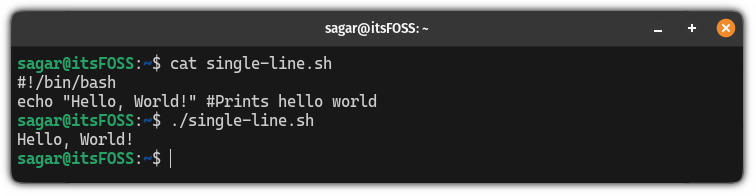
Multi-line comments in bash
As the name suggests, multi-line comments in bash scripting allow you to write comments in multiple lines or prevent executing block of code by putting them in multiline comment section:
- Use # at the begnning of eacj line
- Colon notation (uses colon followed by a single quote)
- Here document (uses << followed by delimiter)
So let's start with the first one.
1. Use # for each line of block comment
This is what I suggest to use if your purpose is to explain part of the script. After all, # is the actual commenting feature.
And this is what many developers use, too.
Suppose you have to explain the purpose of the script, author info or licensing information in the beginning. You can write it like this:
#!/bin/bash
######################################
## This script is used for scanning ##
## local network ##
## Licensed under GPL 2.0 ##
######################################
rest of the bash script code
That's fine when you know how your bash script behaves. If you are debugging a bash script and want to hide part of the script, adding # at the beginning of each line of the required code and then removing them after debugging is a time-consuming task.
The next two sections help you with that.
2. Colon notation
To use the colon notation, you write block comments between : '
and the closing '
as shown here:
#!/bin/bash
: '
This is how you can use colon notation
And this line too will be ignored
'
echo "GOODBYE"
When you execute the above script, it should only print GOODBYE:
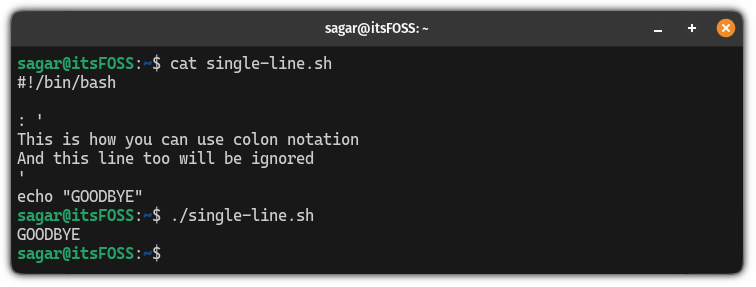
2. Here document
By far, this is the most popular way to write multiline comments in bash which you use <<
followed by a delimiter (a set of characters to specify the start and the end of the comments).
Here's how you use it:
#!/bin/bash
<<DELIMITER
Comment line 1
Comment line 2
DELIMITER
echo "Hello, World!"
Still confused? Here's a simple example:
#!/bin/bash
<<COMMENT
This is a multi-line comment using a here document.
You can add as many lines as you want between <<COMMENT and the terminating keyword.
This block won't be executed by the shell.
COMMENT
echo "Hello, World!"
In the above example, I have used COMMENT
as a delimiter but you can use anything but make sure it stands out from the comment or it will create confusion.
When I executed the above script, it gave me the following output:
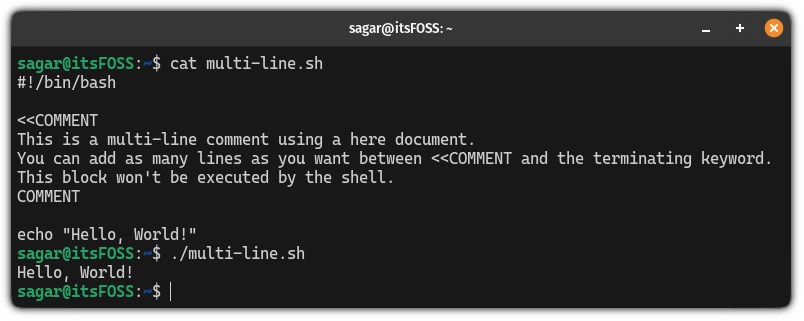
As you can see, it ignored everything inside <<COMMENT
Learn bash from scratch!!
If you are planning to learn bash from scratch or want to skim through all the basics, we made a detailed guide for you:
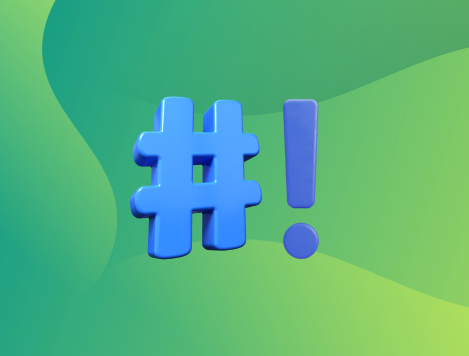
I hope you will find this guide helpful.
Source: It's FOSS