Bash Basics Series #7: If Else Statement
Bash supports if-else statements so that you can use logical reasoning in your shell scripts.
The generic if-else syntax is like this:
if [ expression ]; then
## execute this block if condition is true else go to next
elif [ expression ]; then
## execute this block if condition is true else go to next
else
## if none of the above conditions are true, execute this block
fi
As you can notice:
elif
is used for "else if" kind of condition- The if else conditions always end with
fi
- the use of semicolon
;
andthen
keyword
Before I show the examples of if and else-if, let me share common comparison expressions (also called test conditions) first.
Test conditions
Here are the test condition operators you can use for numeric comparison:
Condition | Equivalent to true when |
---|---|
$a -lt $b | $a < $b ($a is less than $b) |
$a -gt $b | $a > $b ($a is greater than $b) |
$a -le $b | $a <= $b ($a is less or equal than $b) |
$a -ge $b | $a >= $b ($a is greater or equal than $b) |
$a -eq $b | $a is equal to $b |
$a -ne $b | $a is not equal to $b |
If you are comparing strings, you can use these test conditions:
Condition | Equivalent to true when |
---|---|
"$a" = "$b" | $a is same as $b |
"$a" == "$b" | $a is same as $b |
"$a" != "$b" | $a is different from $b |
-z "$a" | $a is empty |
There are also conditions for file type check:
Condition | Equivalent to true when |
---|---|
-f $a | $a is a file |
-d $a | $a is a directory |
-L $a | $a is a link |
Now that you are aware of the various comparison expressions let's see them in action in various examples.
Use if statement in bash
Let's create a script that tells you if a given number is even or not.
Here's my script named even.sh
:
#!/bin/bash
read -p "Enter the number: " num
mod=$(($num%2))
if [ $mod -eq 0 ]; then
echo "Number $num is even"
fi
The modulus operation (%) returns zero when it is perfectly divided by the given number (2 in this case).
Here's what it shows when I run the script:
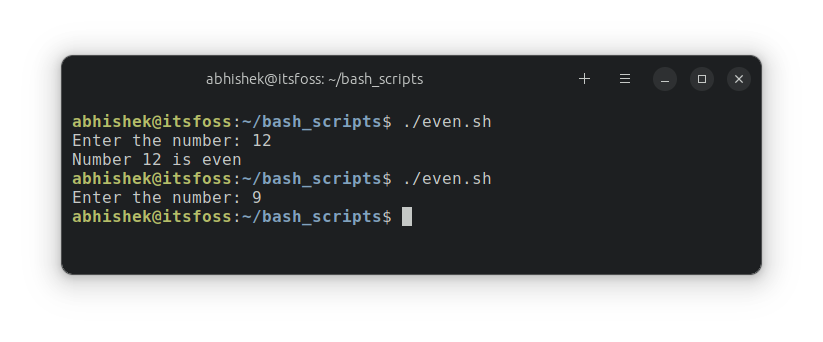
Did you notice that the script tells you when a number is even but it doesn't display anything when the number is odd? Let's improve this script with the use of else.
Use if else statement
Now I add an else statement in the previous script. This way when you get a non-zero modulus (as odd numbers are not divided by 2), it will enter the else block.
#!/bin/bash
read -p "Enter the number: " num
mod=$(($num%2))
if [ $mod -eq 0 ]; then
echo "Number $num is even"
else
echo "Number $num is odd"
fi
Let's run it again with the same numbers:
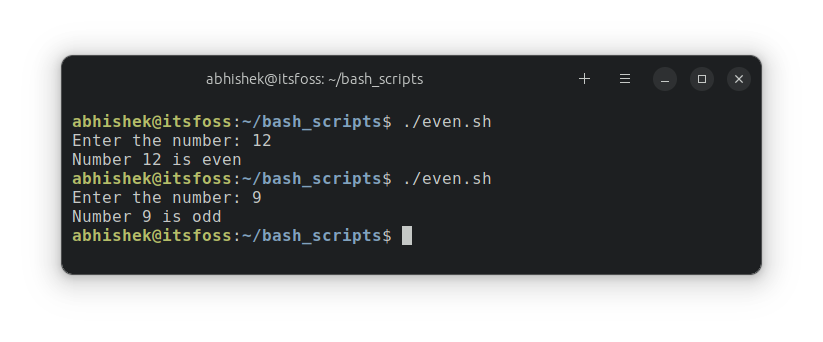
As you can see, the script is better as it also tells you if the number is odd.
Use elif (else if) statement
Here's a script that checks whether the given number is positive or negative. In mathematics, 0 is neither positive nor negative. This script keeps that fact in check as well.
#!/bin/bash
read -p "Enter the number: " num
if [ $num -lt 0 ]; then
echo "Number $num is negative"
elif [ $num -gt 0 ]; then
echo "Number $num is positive"
else
echo "Number $num is zero"
fi
Let me run it to cover all three cases here:
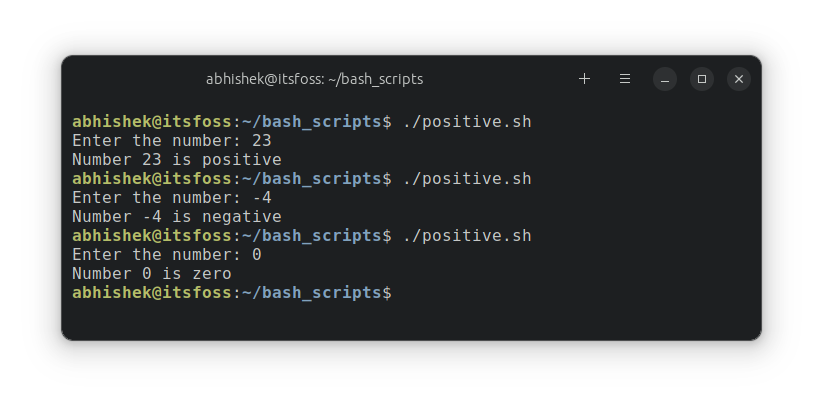
Combine multiple conditions with logical operators
So far, so good. But do you know that you may have multiple conditions in a single by using logical operators like AND (&&), OR (||) etc? It gives you the ability to write complex conditions.
Let's write a script that tells you whether the given year is a leap year or not.
Do you remember the conditions for being a leap year? It should be divided by 4 but if it is divisible by 100, it's not a leap year. However, if it is divisible by 400, it is a leap year.
Here's my script.
#!/bin/bash
read -p "Enter the year: " year
if [[ ($(($year%4)) -eq 0 && $(($year%100)) != 0) || ($(($year%400)) -eq 0) ]]; then
echo "Year $year is leap year"
else
echo "Year $year is normal year"
fi
Verify the script by running it with different data:
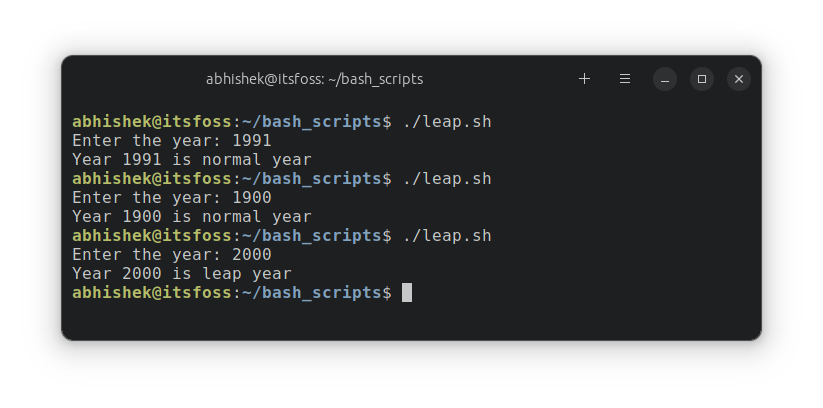
🏋️ Exercise time
Let's do some workout 🙂
Exercise 1: Write a bash shell script that checks the length of the string provided to it as an argument. If no argument is provided, it prints 'empty string'.
Exercise 2: Write a shell script that checks whether a given file exists or not. You can provide the full file path as the argument or use it directly in the script.
Hint: Use -f for file
Exercise 3: Enhance the previous script by checking if the given file is regular file, a directory or a link or if it doesn't exist.
Hint: Use -f, -d and -L
Exercise 3: Write a script that accepts two string arguments. The script should check if the first string contains the second argument as a substring.
Hint: Refer to the previous chapter on bash strings
You may discuss your solution in the Community:
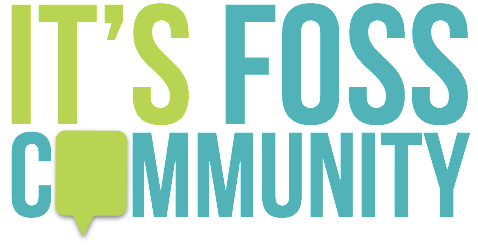
I hope you are enjoying the Bash Basics Series. In the next chapter, you'll learn about using loops in Bash. Keep on bashing!
Source: It's FOSS